Introduction
Accessing reliable financial and economic data is crucial for making informed decisions in investing, research, and policy-making. The Federal Reserve Economic Data (FRED) provides a vast repository of economic data that can be easily accessed and analyzed using Python. This post provides a step-by-step guide to retrieving and visualizing FRED data using Python.
What is FRED?
The FRED is an extensive database maintained by the Federal Reserve Bank of St. Louis. It provides access to a vast array of economic data series, including national, international, public, and private data, covering diverse categories such as banking, employment, population, and more. FRED is widely used by economists, researchers, and analysts to access up-to-date economic data for analysis and forecasting.
How to Use Python to Get Data from FRED
To access FRED data using Python, the fredapi library provides a convenient interface to download data directly from FRED. Here is a step-by-step guide on how to use it:
Step 1: Get Your API Key
Before using fredapi, sign up for an account on the FRED website and obtain an API key. After logging in, find the API key in the account settings.
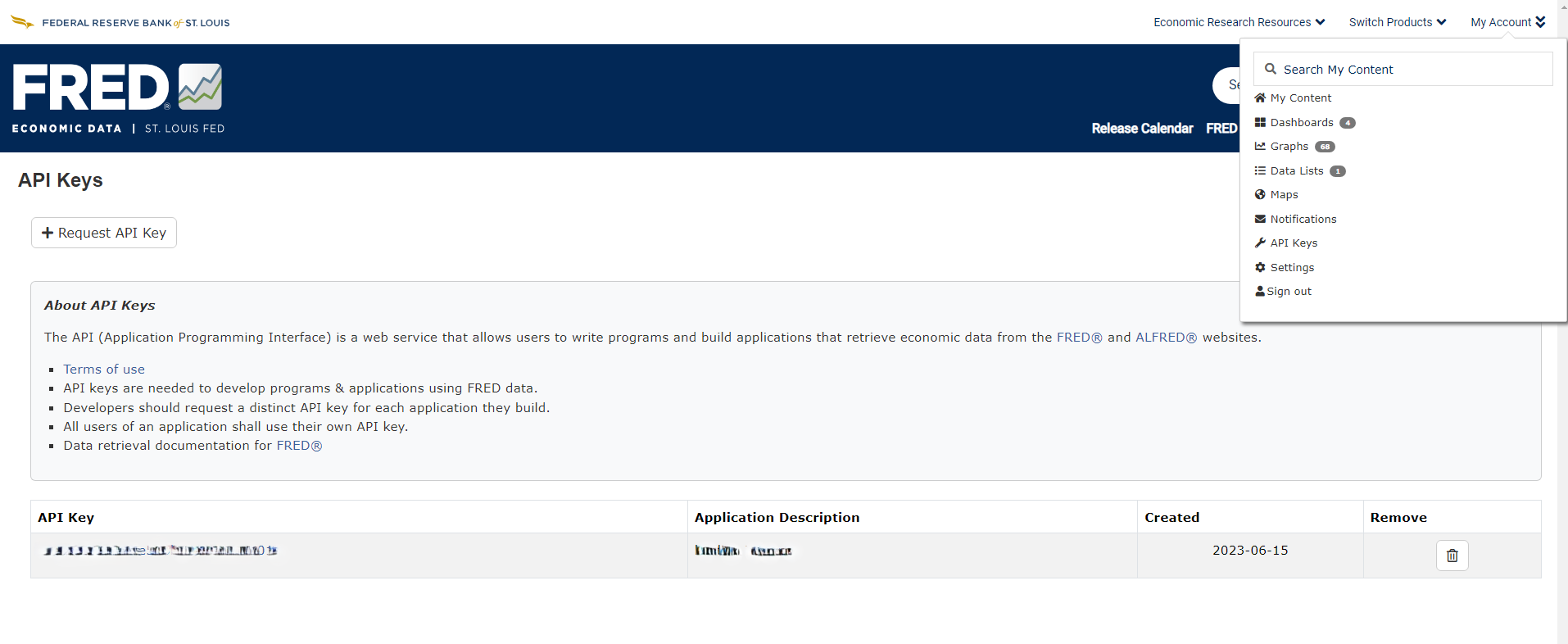
Step 2: Install the Required Libraries
The fredapi and pandas libraries are needed. pandas is used to combine and manipulate the data easily.
pip install fredapi pandas
Step 3: Import the Necessary Libraries
import os
from fredapi import Fred
import pandas as pd
Step 4: Fetch Data from FRED
def get_data_from_fred(data_name):
"""
Fetch data from FRED given a series ID.
Args:
data_name (str): The series ID for the data to fetch from FRED.
Returns:
pd.Series: A pandas Series with the data.
"""
fred = Fred(api_key=os.getenv('FRED_API_KEY'))
try:
data = fred.get_series(data_name)
except Exception as e:
print(f"Error fetching data for {data_name}: {e}")
data = pd.Series()
return data
# List of data series IDs to fetch
data_name_list = ["NASDAQCOM", "NASDAQ100", "DEXUSEU", "DEXJPUS", "T10Y2Y", "T10Y3M", "DGS10",
"DGS2", "DGS3MO"]
# Fetch the data
data_dict = {name: get_data_from_fred(name) for name in data_name_list}
# Create a DataFrame from the dictionary
data = pd.DataFrame(data_dict)
Asset Classes Covered in the Project
Asset Class | FRED ID | Name |
---|---|---|
Equity | NASDAQCOM, NASDAQ100 | NASDAQ Composite Index, NASDAQ 100 Index |
Interest Rate | DGS10, DGS2, DGS3MO | Market Yield on U.S. Treasury Securities at 10-Year Constant Maturity, Market Yield on U.S. Treasury Securities at 2-Year Constant Maturity, Market Yield on U.S. Treasury Securities at 3-Month Constant Maturity |
Term Spread | T10Y2Y, T10Y3M | 10-Year Treasury Constant Maturity Minus 2-Year Treasury Constant Maturity, 10-Year Treasury Constant Maturity Minus 3-Month Treasury Constant Maturity |
Currency | DEXUSEU, DEXJPUS | U.S. Dollars to Euro Spot Exchange Rate, Japanese Yen to U.S. Dollar Spot Exchange Rate |